Merchant API
- Card Numbers Tokenized and Stored for Future Transactions
- Set Up Recurring Payments
- Email and SMS Invoicing
- Cash Discount / Surcharging Features Available
- Level 2 and Level 3 Payment Options
- Dynamic Descriptor Improves Transaction Descriptions on Customer Bank Statements
- Inventory Management
- E-Commerce Integrations with OpenCart, WooCommerce, Magento, Shopify, OsCommerce
- Easy to Use Mobile App Available on IOS and Android
Objective
The objective of an eCommerce payment API (Application Programming Interface) is to facilitate and streamline the process of handling online payments for eCommerce businesses. These APIs serve as a bridge between the online store and payment processing systems, allowing for the secure and efficient transfer of funds. Here are the key objectives of an eCommerce payment API:
Payment Processing: The primary objective is to enable the processing of online payments, including credit/debit card payments, digital wallet transactions, bank transfers, and other payment methods.
Security: Ensure the security of payment transactions by implementing encryption, tokenization, and other security measures to protect sensitive customer and payment data.
Scalability: Facilitate scalability so that businesses can handle a growing number of transactions without significant issues or disruptions.
Real-Time Processing: Process payments in real-time or near real-time, providing immediate feedback to customers about the success or failure of their transactions.
Compliance: Ensure that the payment API complies with industry standards and regulations, such as Payment Card Industry Data Security Standard (PCI DSS), to protect customer data and maintain trust.
Integration: Make it easy for developers to integrate the payment API into various eCommerce platforms, content management systems, and mobile apps.
Customer Support: Offer customer support and assistance for any payment-related issues or inquiries.
Connections
The following are the sandbox credentials, Email: [email protected] for production credentials
- Protocol: Https
- Port: 4430
- Method: POST
- URL: URL
- Please email to [email protected] to get the following auth credentials
- APP_ID
- APP_KEY
Transaction Flow
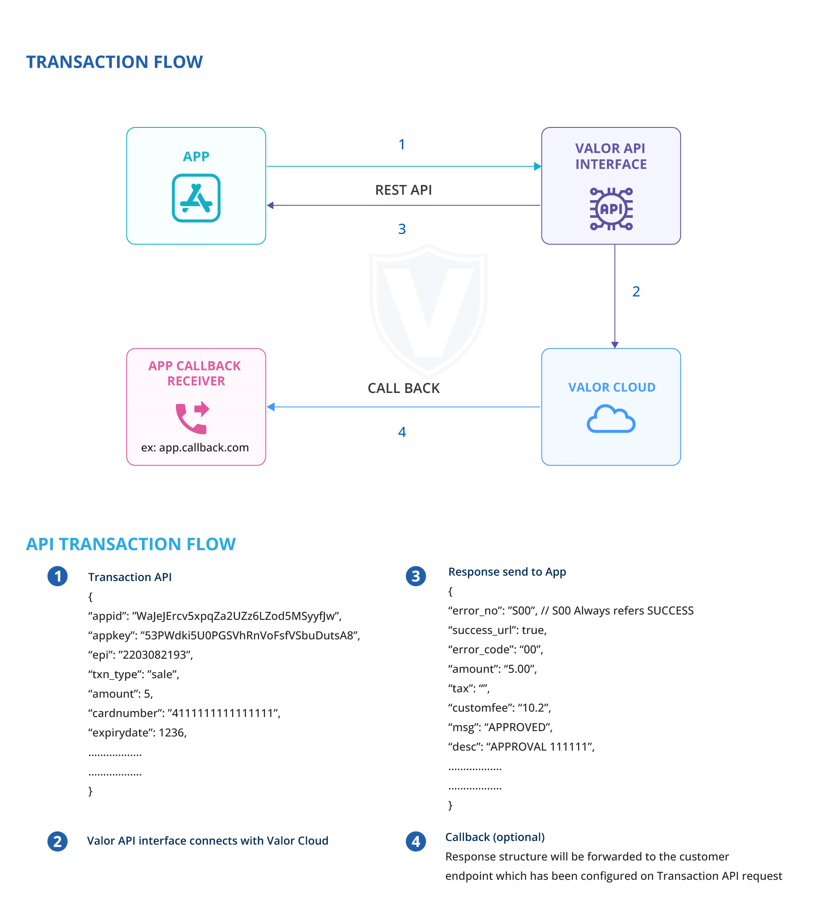
Direct Sale API
This API enables the secure and seamless handling of payments, including credit & debit card transactions, for products or services offered for sale on their website.
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?sale=', [
'body' => '{"appid":"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw","appkey":"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8","epi":"2203082193","txn_type":"sale","amount":5,"cardnumber":"4111111111111111","expirydate":1236,"cvv":999,"cardholdername":"Michael Jordan","invoicenumber":"inv0001","orderdescription":"king size bed 10x12","surchargeAmount":10.2,"surchargeIndicator":1,"address1":"2 Jericho Plz","city":"Jericho","state":"NY","shipping_country":"US","billing_country":"US","zip":"50001"}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?sale="
payload = {
"appid": "WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw",
"appkey": "53PWdki5U0PGSVhRnVoFsfVSbuDutsA8",
"epi": "2203082193",
"txn_type": "sale",
"amount": 5,
"cardnumber": "4111111111111111",
"expirydate": 1236,
"cvv": 999,
"cardholdername": "Michael Jordan",
"invoicenumber": "inv0001",
"orderdescription": "king size bed 10x12",
"surchargeAmount": 10.2,
"surchargeIndicator": 1,
"address1": "2 Jericho Plz",
"city": "Jericho",
"state": "NY",
"shipping_country": "US",
"billing_country": "US",
"zip": "50001"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#fkfd1tylnog14l9');
sdk.directSale({
appid: 'WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw',
appkey: '53PWdki5U0PGSVhRnVoFsfVSbuDutsA8',
epi: '2203082193',
txn_type: 'sale',
amount: 5,
cardnumber: '4111111111111111',
expirydate: 1236,
cvv: 999,
cardholdername: 'Michael Jordan',
invoicenumber: 'inv0001',
orderdescription: 'king size bed 10x12',
surchargeAmount: 10.2,
surchargeIndicator: 1,
address1: '2 Jericho Plz',
city: 'Jericho',
state: 'NY',
shipping_country: 'US',
billing_country: 'US',
zip: '50001'
}, {sale: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?sale=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"sale\",\"amount\":5,\"cardnumber\":\"4111111111111111\",\"expirydate\":1236,\"cvv\":999,\"cardholdername\":\"Michael Jordan\",\"invoicenumber\":\"inv0001\",\"orderdescription\":\"king size bed 10x12\",\"surchargeAmount\":10.2,\"surchargeIndicator\":1,\"address1\":\"2 Jericho Plz\",\"city\":\"Jericho\",\"state\":\"NY\",\"shipping_country\":\"US\",\"billing_country\":\"US\",\"zip\":\"50001\"}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?sale=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"sale\",\"amount\":5,\"cardnumber\":\"4111111111111111\",\"expirydate\":1236,\"cvv\":999,\"cardholdername\":\"Michael Jordan\",\"invoicenumber\":\"inv0001\",\"orderdescription\":\"king size bed 10x12\",\"surchargeAmount\":10.2,\"surchargeIndicator\":1,\"address1\":\"2 Jericho Plz\",\"city\":\"Jericho\",\"state\":\"NY\",\"shipping_country\":\"US\",\"billing_country\":\"US\",\"zip\":\"50001\"}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?sale="
payload := strings.NewReader("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"sale\",\"amount\":5,\"cardnumber\":\"4111111111111111\",\"expirydate\":1236,\"cvv\":999,\"cardholdername\":\"Michael Jordan\",\"invoicenumber\":\"inv0001\",\"orderdescription\":\"king size bed 10x12\",\"surchargeAmount\":10.2,\"surchargeIndicator\":1,\"address1\":\"2 Jericho Plz\",\"city\":\"Jericho\",\"state\":\"NY\",\"shipping_country\":\"US\",\"billing_country\":\"US\",\"zip\":\"50001\"}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?sale=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"sale\",\"amount\":5,\"cardnumber\":\"4111111111111111\",\"expirydate\":1236,\"cvv\":999,\"cardholdername\":\"Michael Jordan\",\"invoicenumber\":\"inv0001\",\"orderdescription\":\"king size bed 10x12\",\"surchargeAmount\":10.2,\"surchargeIndicator\":1,\"address1\":\"2 Jericho Plz\",\"city\":\"Jericho\",\"state\":\"NY\",\"shipping_country\":\"US\",\"billing_country\":\"US\",\"zip\":\"50001\"}"
response = http.request(request)
puts response.read_body
{
"error_no": "S00",
"success_url": true,
"error_code": "00",
"amount": "5.00",
"tax": "",
"customfee": "10.2",
"msg": "APPROVED",
"desc": "APPROVAL 111111 ",
"additional_info": null,
"clerk_id": null,
"clerk_name": null,
"clerk_label": null,
"additionalKeyOne": null,
"additionalKeyTwo": null,
"additionalValueOne": null,
"additionalValueTwo": null,
"approval_code": "111111",
"rrn": "329112751532",
"txnid": "5239902",
"tran_no": 14,
"stan": 133,
"is_partial_approve": 0,
"partial_amount": "000000000500",
"pan": "XXXX1111",
"card_type": null,
"phone_number": "",
"email_id": "",
"zip": null,
"card_holder_name": "Michael Jordan",
"expiry_date": "12/36",
"address": "",
"epi": "2203082193",
"channel": "ECOMM",
"token": "5039558440E9B224473C7EE673560859C556AA35",
"card_brand": "Visa",
"netamt": 15.2
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory sale, auth sale or auth ('sale' - Amount of the purchase will be deducted from the cardholder's bank account and 'auth' -
it will be held (authorized) for a certain period of time/days)
amount Numeric 10 Mandatory 100.50 Transaction amount (Maximum amount 99,999.99)
cardnumber Numeric 19 Mandatory 4012881888818888 Customer card number 15 to 19 digits
expirydate date 5 Mandatory 1225 Card expiry date, Accepted expiry MMYY or MM/YY
cvv Numeric 4 Optional 523 or 4251 3 or 4 digits number
cardholdername String 25 Optional John Smith Name of the cardholder - Alphabet
invoicenumber String 12 Optional inv0021 Invoice number is a unique number assigned (Alphanumeric)
orderdescription String 50 Optional Ex.Comments Add the order description - Optional (Alphanumeric)
surchargeAmount Numeric 4 Optional 4.00 Additional fee of surcharge amount - Currency $ (Eg: 10.00)
surchargeIndicator Numeric 1 Mandatory 0 or 1 The value '0' represents a transaction being ran on the traditional MID with no custom fee added, while
'1' represents a transaction being ran on the cash discounting MID with the custom fee added
phone Number 10 Optional 8965342569 Customer phone number - 10 digit
address1 String 100 Optional 2nd Avenue Shipping address should be max 100 chars - Alphanumeric
address2 String 100 Optional 2nd Avenue Billing address should be max 100 chars - Alphanumeric
city Numeric 50 Optional New York Address city - 15 chars
state String 25 Optional NY, CA Shipping address state (2 chars capital)
shipping_country String 25 Optinonal US Shipping country should be mention default set us US
billing_country String 25 Optinonal US Billing country should be mention default set us US
zip String 5 Optional 10001, 90254 Zip code should be 5 digits
email String 50 Optional [email protected] Customer email id - Varchar 255
Direct Sale Token
A unique payment link is generated through the Direct Sale Token API, which can be sent to the customer via their phone number and email. The customer can then use this link to proceed with the payment.
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?pagesale=', [
'body' => '{"appid":"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw","appkey":"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8","epi":"2203082193","txn_type":"sale","amount":5,"invoicenumber":"inv0001","orderdescription":"king size bed 10x12","surcharge":10.2,"tax":12.34,"epage":1,"ignore_surcharge_calc":0,"token":"ED72E29E309E6B86297AAF3BA18FE7B6F81D6E94"}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?pagesale="
payload = {
"appid": "WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw",
"appkey": "53PWdki5U0PGSVhRnVoFsfVSbuDutsA8",
"epi": "2203082193",
"txn_type": "sale",
"amount": 5,
"invoicenumber": "inv0001",
"orderdescription": "king size bed 10x12",
"surcharge": 10.2,
"tax": 12.34,
"epage": 1,
"ignore_surcharge_calc": 0,
"token": "ED72E29E309E6B86297AAF3BA18FE7B6F81D6E94"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#fkfd1tylnog14l9');
sdk.directSaleToken1({
appid: 'WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw',
appkey: '53PWdki5U0PGSVhRnVoFsfVSbuDutsA8',
epi: '2203082193',
txn_type: 'sale',
amount: 5,
invoicenumber: 'inv0001',
orderdescription: 'king size bed 10x12',
surcharge: 10.2,
tax: 12.34,
epage: 1,
ignore_surcharge_calc: 0,
token: 'ED72E29E309E6B86297AAF3BA18FE7B6F81D6E94'
}, {pagesale: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?pagesale=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"sale\",\"amount\":5,\"invoicenumber\":\"inv0001\",\"orderdescription\":\"king size bed 10x12\",\"surcharge\":10.2,\"tax\":12.34,\"epage\":1,\"ignore_surcharge_calc\":0,\"token\":\"ED72E29E309E6B86297AAF3BA18FE7B6F81D6E94\"}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?pagesale=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"sale\",\"amount\":5,\"invoicenumber\":\"inv0001\",\"orderdescription\":\"king size bed 10x12\",\"surcharge\":10.2,\"tax\":12.34,\"epage\":1,\"ignore_surcharge_calc\":0,\"token\":\"ED72E29E309E6B86297AAF3BA18FE7B6F81D6E94\"}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?pagesale="
payload := strings.NewReader("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"sale\",\"amount\":5,\"invoicenumber\":\"inv0001\",\"orderdescription\":\"king size bed 10x12\",\"surcharge\":10.2,\"tax\":12.34,\"epage\":1,\"ignore_surcharge_calc\":0,\"token\":\"ED72E29E309E6B86297AAF3BA18FE7B6F81D6E94\"}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?pagesale=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"sale\",\"amount\":5,\"invoicenumber\":\"inv0001\",\"orderdescription\":\"king size bed 10x12\",\"surcharge\":10.2,\"tax\":12.34,\"epage\":1,\"ignore_surcharge_calc\":0,\"token\":\"ED72E29E309E6B86297AAF3BA18FE7B6F81D6E94\"}"
response = http.request(request)
puts response.read_body
{
"error_no": "S00",
"url": "https://securelink-staging.valorpaytech.com:4430//?uid=ddVA9cF2EwTViC3ZmbUWHfS8BIbe6sBt&redirect=1",
"uid": "ddVA9cF2EwTViC3ZmbUWHfS8BIbe6sBt"
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory sale, auth sale or auth ('sale' - Amount of the purchase will be deducted from the cardholder's bank account and 'auth' -
it will be held (authorized) for a certain period of time/days)
amount Numeric 10 Mandatory 100.50 Transaction amount (Maximum amount 99,999.99)
invoicenumber String 12 Optional inv0021 Invoice number is a unique number assigned (Alphanumeric)
orderdescription String 50 Optional Ex.Comments Add the order description - Optional (Alphanumeric)
surcharge Numeric 4 Optional 4.00 Additional fee of surcharge amount - Currency $ (Eg: 10.00)
phone Number 10 Optional 8965342569 Customer phone number - 10 digit
email String 50 Optional [email protected] Customer email id - Varchar 255
tax Numeric 10 Optinonal 2.0 Tax amount - Currency $
epage Numeric 1 Mandatory 1 Should be set always 1
redirect_url String 100 Optinonal E: shopify.com Merchants call back url (This URL is where the merchant's system can receive information or updates
about a transaction, such as payment confirmation, order status, or other relevant data.)
ignore_surcharge_calc Numeric 1 Mandatory 0 or 1 Set value 0 or 1 (1 - It will calculte the surcharge value from here and 0 - Fee will be calculate
from merchant portal)
success_url String 100 Optinonal Ex: recipt_url Successful transaction, page redirect to success page
failure_url String 100 Optinonal Ex: recipt_url Failed transaction, page redirect to failed page
token String 50 Mandatory ED72E29E309E6B86297AAF3BA18FE778 Token get from direct sale API
Hosted Page Sale
A unique payment link is generated through the Direct Sale Token API, which can be sent to the customer via their phone number and email. The customer can then use this link to proceed with the payment.
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?pagesale=', [
'body' => '{"appid":"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw","appkey":"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8","epi":"2203082193","txn_type":"sale","amount":5,"invoicenumber":"inv0001","orderdescription":"king size bed 10x12","surcharge":10.2,"tax":12.34,"epage":1,"ignore_surcharge_calc":0,"phone":"5248785869","email":"[email protected]"}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?pagesale="
payload = {
"appid": "WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw",
"appkey": "53PWdki5U0PGSVhRnVoFsfVSbuDutsA8",
"epi": "2203082193",
"txn_type": "sale",
"amount": 5,
"invoicenumber": "inv0001",
"orderdescription": "king size bed 10x12",
"surcharge": 10.2,
"tax": 12.34,
"epage": 1,
"ignore_surcharge_calc": 0,
"phone": "5248785869",
"email": "[email protected]"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#fkfd1tylnog14l9');
sdk.directSaleToken1({
appid: 'WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw',
appkey: '53PWdki5U0PGSVhRnVoFsfVSbuDutsA8',
epi: '2203082193',
txn_type: 'sale',
amount: 5,
invoicenumber: 'inv0001',
orderdescription: 'king size bed 10x12',
surcharge: 10.2,
tax: 12.34,
epage: 1,
ignore_surcharge_calc: 0,
phone: '5248785869',
email: '[email protected]'
}, {pagesale: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?pagesale=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"sale\",\"amount\":5,\"invoicenumber\":\"inv0001\",\"orderdescription\":\"king size bed 10x12\",\"surcharge\":10.2,\"tax\":12.34,\"epage\":1,\"ignore_surcharge_calc\":0,\"phone\":\"5248785869\",\"email\":\"[email protected]\"}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?pagesale=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"sale\",\"amount\":5,\"invoicenumber\":\"inv0001\",\"orderdescription\":\"king size bed 10x12\",\"surcharge\":10.2,\"tax\":12.34,\"epage\":1,\"ignore_surcharge_calc\":0,\"phone\":\"5248785869\",\"email\":\"[email protected]\"}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?pagesale="
payload := strings.NewReader("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"sale\",\"amount\":5,\"invoicenumber\":\"inv0001\",\"orderdescription\":\"king size bed 10x12\",\"surcharge\":10.2,\"tax\":12.34,\"epage\":1,\"ignore_surcharge_calc\":0,\"phone\":\"5248785869\",\"email\":\"[email protected]\"}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?pagesale=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"sale\",\"amount\":5,\"invoicenumber\":\"inv0001\",\"orderdescription\":\"king size bed 10x12\",\"surcharge\":10.2,\"tax\":12.34,\"epage\":1,\"ignore_surcharge_calc\":0,\"phone\":\"5248785869\",\"email\":\"[email protected]\"}"
response = http.request(request)
puts response.read_body
{
"error_no": "S00",
"url": "https://securelink-staging.valorpaytech.com:4430//?uid=rdAEM8NyAbYmXwG3tXdH6oYr6HXAslvA&redirect=1",
"uid": "rdAEM8NyAbYmXwG3tXdH6oYr6HXAslvA"
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory sale, auth sale or auth ('sale' - Amount of the purchase will be deducted from the cardholder's bank account and 'auth' -
it will be held (authorized) for a certain period of time/days)
amount Numeric 10 Mandatory 100.50 Transaction amount (Maximum amount 99,999.99)
invoicenumber String 12 Optional inv0021 Invoice number is a unique number assigned (Alphanumeric)
orderdescription String 50 Optional Ex.Comments Add the order description - Optional (Alphanumeric)
surcharge Numeric 4 Optional 4.00 Additional fee of surcharge amount - Currency $ (Eg: 10.00)
phone Number 10 Optional 8965342569 Customer phone number - 10 digit
email String 50 Optional [email protected] Customer email id - Varchar 255
tax Numeric 10 Optinonal 2.0 Tax amount - Currency $
epage Numeric 1 Mandatory 1 Should be set always 1
redirect_url String 100 Optinonal E: shopify.com Merchants call back url (This URL is where the merchant's system can receive information or updates
about a transaction, such as payment confirmation, order status, or other relevant data.)
ignore_surcharge_calc Numeric 1 Mandatory 0 or 1 Set value 0 or 1 (1 - It will calculte the surcharge value from here and 0 - Fee will be calculate
from merchant portal)
success_url String 100 Optinonal Ex: recipt_url Successful transaction, page redirect to success page
failure_url String 100 Optinonal Ex: recipt_url Failed transaction, page redirect to failed page
Merchant Hosted Page Sale
Proceeding with the sale using the hosted page sale API uid, the merchant can complete the transaction through the provided API.
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?epage=', [
'body' => '{"uid_mode":1,"cardnumber":4111111111111111,"expirydate":"1236","cvv":"999","epage":1,"uid":"PQyj0wrzhjWP4YPA8b3FQcPDiUYDEqjH","phone":"5875968574","email":"[email protected]"}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?epage="
payload = {
"uid_mode": 1,
"cardnumber": 4111111111111111,
"expirydate": "1236",
"cvv": "999",
"epage": 1,
"uid": "PQyj0wrzhjWP4YPA8b3FQcPDiUYDEqjH",
"phone": "5875968574",
"email": "[email protected]"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#fkfd1tylnog14l9');
sdk.merchantHostedPageSale({
uid_mode: 1,
cardnumber: 4111111111111111,
expirydate: '1236',
cvv: '999',
epage: 1,
uid: 'PQyj0wrzhjWP4YPA8b3FQcPDiUYDEqjH',
phone: '5875968574',
email: '[email protected]'
}, {epage: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?epage=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"uid_mode\":1,\"cardnumber\":4111111111111111,\"expirydate\":\"1236\",\"cvv\":\"999\",\"epage\":1,\"uid\":\"PQyj0wrzhjWP4YPA8b3FQcPDiUYDEqjH\",\"phone\":\"5875968574\",\"email\":\"[email protected]\"}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?epage=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"uid_mode\":1,\"cardnumber\":4111111111111111,\"expirydate\":\"1236\",\"cvv\":\"999\",\"epage\":1,\"uid\":\"PQyj0wrzhjWP4YPA8b3FQcPDiUYDEqjH\",\"phone\":\"5875968574\",\"email\":\"[email protected]\"}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?epage="
payload := strings.NewReader("{\"uid_mode\":1,\"cardnumber\":4111111111111111,\"expirydate\":\"1236\",\"cvv\":\"999\",\"epage\":1,\"uid\":\"PQyj0wrzhjWP4YPA8b3FQcPDiUYDEqjH\",\"phone\":\"5875968574\",\"email\":\"[email protected]\"}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?epage=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"uid_mode\":1,\"cardnumber\":4111111111111111,\"expirydate\":\"1236\",\"cvv\":\"999\",\"epage\":1,\"uid\":\"PQyj0wrzhjWP4YPA8b3FQcPDiUYDEqjH\",\"phone\":\"5875968574\",\"email\":\"[email protected]\"}"
response = http.request(request)
puts response.read_body
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=0.9,maximum-scale=0.9 shrink-to-fit=yes">
<title>Valor paytech</title>
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto|Varela+Round">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css">
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css">
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/umd/popper.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/js/bootstrap.min.js"></script>
<style>
body {
font-family: 'Varela Round', sans-serif;
}
.modal-confirm {
color: #636363;
width: auto;
font-size: 14px;
}
.modal-confirm .modal-content {
padding: 20px;
border-radius: 5px;
border: none;
}
.modal-confirm .modal-header {
border-bottom: none;
position: relative;
}
.modal-confirm h4 {
text-align: center;
font-size: 26px;
margin: 30px 0 -15px;
}
.modal-confirm .form-control, .modal-confirm .btn {
min-height: 5px;
border-radius: 3px;
}
.modal-confirm .close {
position: absolute;
top: -5px;
right: -40px;
}
.modal-confirm .modal-footer {
border: none;
text-align: center;
border-radius: 5px;
font-size: 13px;
}
.modal-confirm .icon-box {
color: #fff;
position: absolute;
margin: 0 auto;
left: 0;
right: 0;
top: -70px;
width: 95px;
height: 95px;
border-radius: 50%;
z-index: 9;
background: #82ce34;
padding: 15px;
text-align: center;
box-shadow: 0px 2px 2px rgba(0, 0, 0, 0.1);
}
.modal-confirm .icon-box i {
font-size: 58px;
position: relative;
top: 3px;
}
.modal-confirm.modal-dialog {
margin-top: 200px;
}
.modal-confirm .btn {
color: #fff;
border-radius: 4px;
background: #82ce34;
text-decoration: none;
transition: all 0.4s;
line-height: normal;
border: none;
}
.modal-confirm .btn:hover, .modal-confirm .btn:focus {
background: #6fb32b;
outline: none;
}
.trigger-btn {
display: inline-block;
margin: 100px auto;
}
</style>
</head>
<body>
<!-- Modal HTML -->
<div id="myModal123" class="">
<div class="modal-dialog modal-confirm">
<div class="modal-content">
<div class="modal-header">
<div class="icon-box">
<i class="material-icons"></i>
</div>
<h4 class="modal-title w-100">APPROVED</h4>
</div>
<div class="modal-body">
<p class="text-center">Your transaction has been successfully processed.</p>
</div>
</div>
</div>
</div>
</body>
</html>
Field Type Length Mandatory/Optional Example Value Description
uid_mode Numeric 1 Mandatory 1 Should be set as 1
uid String 32 Mandatory PQyj0wrzhjWP4YPA8b3FQcPDiUYDEqjH UID can be generated from Hosted Page Sale API
cardnumber Numeric 19 Mandatory 4012881888818888 Customer card number 15 to 19 digits
expirydate date 5 Mandatory 1225 Card expiry date, Accepted expiry MMYY or MM/YY
cvv Numeric 4 Optional 523 or 4251 3 or 4 digits number
phone Number 10 Optional 8965342569 Customer phone number - 10 digit
email String 50 Optional [email protected] Customer email id - Varchar 255
epage Numeric 1 Mandatory 1 Should be set always 1
redirect_url String 100 Optinonal Ex: shopify.com Merchants call back url (This URL is where the merchant's system can receive information or updates
about a transaction, such as payment confirmation, order status, or other relevant data.)
Refund Offset Sale
The "Refund Offset API" is utilized to process a refund for a payment by referencing the transaction ID, authorization code, RRN (Retrieval Reference Number), and a token associated with the original sale.
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?refund=', [
'body' => '{"appid":"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw","appkey":"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8","epi":"2203082193","txn_type":"refund","amount":5,"surchargeIndicator":1,"sale_refund":"1","rrn":"329210751122","auth_code":"111111","ref_txn_id":"5244370","token":"6244564890801A3154FA3706E02FD96C12988FD6"}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?refund="
payload = {
"appid": "WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw",
"appkey": "53PWdki5U0PGSVhRnVoFsfVSbuDutsA8",
"epi": "2203082193",
"txn_type": "refund",
"amount": 5,
"surchargeIndicator": 1,
"sale_refund": "1",
"rrn": "329210751122",
"auth_code": "111111",
"ref_txn_id": "5244370",
"token": "6244564890801A3154FA3706E02FD96C12988FD6"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#fkfd1tylnog14l9');
sdk.directSaleCopy({
appid: 'WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw',
appkey: '53PWdki5U0PGSVhRnVoFsfVSbuDutsA8',
epi: '2203082193',
txn_type: 'refund',
amount: 5,
surchargeIndicator: 1,
sale_refund: '1',
rrn: '329210751122',
auth_code: '111111',
ref_txn_id: '5244370',
token: '6244564890801A3154FA3706E02FD96C12988FD6'
}, {refund: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?refund=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"refund\",\"amount\":5,\"surchargeIndicator\":1,\"sale_refund\":\"1\",\"rrn\":\"329210751122\",\"auth_code\":\"111111\",\"ref_txn_id\":\"5244370\",\"token\":\"6244564890801A3154FA3706E02FD96C12988FD6\"}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?refund=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"refund\",\"amount\":5,\"surchargeIndicator\":1,\"sale_refund\":\"1\",\"rrn\":\"329210751122\",\"auth_code\":\"111111\",\"ref_txn_id\":\"5244370\",\"token\":\"6244564890801A3154FA3706E02FD96C12988FD6\"}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?refund="
payload := strings.NewReader("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"refund\",\"amount\":5,\"surchargeIndicator\":1,\"sale_refund\":\"1\",\"rrn\":\"329210751122\",\"auth_code\":\"111111\",\"ref_txn_id\":\"5244370\",\"token\":\"6244564890801A3154FA3706E02FD96C12988FD6\"}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?refund=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"refund\",\"amount\":5,\"surchargeIndicator\":1,\"sale_refund\":\"1\",\"rrn\":\"329210751122\",\"auth_code\":\"111111\",\"ref_txn_id\":\"5244370\",\"token\":\"6244564890801A3154FA3706E02FD96C12988FD6\"}"
response = http.request(request)
puts response.read_body
{
"error_no": "S00",
"error_code": "00",
"amount": "5.00",
"msg": "APPROVED",
"desc": "APPROVAL 111111 ",
"additional_info": null,
"epi": "2203082193",
"channel": "ECOMM",
"rrn": "329210751123",
"txnid": "5244398"
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory refund Transaction type should be set as refund
amount Numeric 10 Mandatory 100.50 Transaction amount (Maximum amount 99,999.99)
surchargeIndicator Numeric 1 Mandatory 0 or 1 The value '0' represents a transaction being ran on the traditional MID with no custom fee added, while
'1' represents a transaction being ran on the cash discounting MID with the custom fee added
ref_txn_id String 20 Mandatory 5244370 A unique response transaction id from the response of a sale transaction
sale_refund Numeric 1 Mandatory 1 Should be set as value 1
auth_code String 8 Mandatory TAS12455 Approval code response from sale transaction (Alphanumeric)
rrn String 15 Mandatory 32921075112 RRN number from sale txn response (Numeric)
token String 50 Mandatory 6244564890801A3154FA3706E02FD96C1 Card token, received from sale, use it only if you need to change the card number
Transaction List API
The "Transaction List API" is employed to retrieve transactions based on criteria such as device type, transaction status, source, and card type.
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?txnfetch=', [
'body' => '{"appid":"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw","appkey":"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8","epi":"2203082193","txn_type":"txnfetch","date_filter":0,"transaction_status":"ALL","devices":"ALL","limit":200,"offset":0,"transaction_type":"ALL","processor":"ALL","card_type":"ALL","source":"ALL"}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?txnfetch="
payload = {
"appid": "WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw",
"appkey": "53PWdki5U0PGSVhRnVoFsfVSbuDutsA8",
"epi": "2203082193",
"txn_type": "txnfetch",
"date_filter": 0,
"transaction_status": "ALL",
"devices": "ALL",
"limit": 200,
"offset": 0,
"transaction_type": "ALL",
"processor": "ALL",
"card_type": "ALL",
"source": "ALL"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#fkfd1tylnog14l9');
sdk.transactionListApi({
appid: 'WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw',
appkey: '53PWdki5U0PGSVhRnVoFsfVSbuDutsA8',
epi: '2203082193',
txn_type: 'txnfetch',
date_filter: 0,
transaction_status: 'ALL',
devices: 'ALL',
limit: 200,
offset: 0,
transaction_type: 'ALL',
processor: 'ALL',
card_type: 'ALL',
source: 'ALL'
}, {txnfetch: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?txnfetch=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"txnfetch\",\"date_filter\":0,\"transaction_status\":\"ALL\",\"devices\":\"ALL\",\"limit\":200,\"offset\":0,\"transaction_type\":\"ALL\",\"processor\":\"ALL\",\"card_type\":\"ALL\",\"source\":\"ALL\"}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?txnfetch=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"txnfetch\",\"date_filter\":0,\"transaction_status\":\"ALL\",\"devices\":\"ALL\",\"limit\":200,\"offset\":0,\"transaction_type\":\"ALL\",\"processor\":\"ALL\",\"card_type\":\"ALL\",\"source\":\"ALL\"}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?txnfetch="
payload := strings.NewReader("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"txnfetch\",\"date_filter\":0,\"transaction_status\":\"ALL\",\"devices\":\"ALL\",\"limit\":200,\"offset\":0,\"transaction_type\":\"ALL\",\"processor\":\"ALL\",\"card_type\":\"ALL\",\"source\":\"ALL\"}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?txnfetch=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"txnfetch\",\"date_filter\":0,\"transaction_status\":\"ALL\",\"devices\":\"ALL\",\"limit\":200,\"offset\":0,\"transaction_type\":\"ALL\",\"processor\":\"ALL\",\"card_type\":\"ALL\",\"source\":\"ALL\"}"
response = http.request(request)
puts response.read_body
{
"number_of_records": 62,
"data": [
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5244398",
"TIME": "06:36 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329210751123",
"TXN_TYPE": "REFUND",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michael Jordan",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "012",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "20",
"STAN_NO": "405",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 10:36:27",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5244370",
"TIME": "06:34 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329210751122",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michael Jordan",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "1020",
"NET_AMOUNT": "1520",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "19",
"STAN_NO": "401",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "king size bed 10x12",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 10:34:37",
"BILLING_STREET_NO": "2 Jericho Plz",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "50001",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5244054",
"TIME": "06:10 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329210751108",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "18",
"STAN_NO": "339",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 10:10:17",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5243934",
"TIME": "06:01 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329210751103",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michael Jordan",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "1020",
"NET_AMOUNT": "1520",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "17",
"STAN_NO": "332",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "king size bed 10x12",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 10:01:26",
"BILLING_STREET_NO": "2 Jericho Plz",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "50001",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5243334",
"TIME": "04:38 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329208751089",
"TXN_TYPE": "REFUND",
"CARD_SCHEME": "",
"PAN": "",
"RESPONSE_CODE": "12",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "012",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "17",
"STAN_NO": "295",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "Original transaction not found",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 08:38:11",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5243306",
"TIME": "04:32 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329208751089",
"TXN_TYPE": "REFUND",
"CARD_SCHEME": "",
"PAN": "",
"RESPONSE_CODE": "12",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "012",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "17",
"STAN_NO": "337",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "Original transaction not found",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 08:32:31",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5243302",
"TIME": "04:32 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329208751089",
"TXN_TYPE": "REFUND",
"CARD_SCHEME": "",
"PAN": "",
"RESPONSE_CODE": "12",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "012",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "17",
"STAN_NO": "336",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "Original transaction not found",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 08:32:29",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5243298",
"TIME": "04:32 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329208751089",
"TXN_TYPE": "REFUND",
"CARD_SCHEME": "",
"PAN": "",
"RESPONSE_CODE": "12",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "012",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "17",
"STAN_NO": "291",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "Original transaction not found",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 08:32:09",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5243294",
"TIME": "04:31 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329208751089",
"TXN_TYPE": "REFUND",
"CARD_SCHEME": "",
"PAN": "",
"RESPONSE_CODE": "12",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "012",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "17",
"STAN_NO": "290",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "Original transaction not found",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 08:31:54",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5243290",
"TIME": "04:31 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329208751089",
"TXN_TYPE": "REFUND",
"CARD_SCHEME": "",
"PAN": "",
"RESPONSE_CODE": "12",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "012",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "17",
"STAN_NO": "335",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "Original transaction not found",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 08:31:39",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5243282",
"TIME": "04:28 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329208751089",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michael Jordan",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "1020",
"NET_AMOUNT": "1520",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "16",
"STAN_NO": "334",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "king size bed 10x12",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "1",
"TXN_ORIG_DATE": "2023-10-19 08:28:53",
"BILLING_STREET_NO": "2 Jericho Plz",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "50001",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5242722",
"TIME": "02:50 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329206751067",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "17",
"NET_AMOUNT": "1751",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "15",
"STAN_NO": "299",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 06:50:47",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5242466",
"TIME": "02:21 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329206751060",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "17",
"NET_AMOUNT": "1751",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "14",
"STAN_NO": "278",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 06:21:45",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5242434",
"TIME": "02:18 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329206751056",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "13",
"STAN_NO": "277",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 06:18:52",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5242382",
"TIME": "02:14 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329206751052",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "12",
"STAN_NO": "271",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 06:14:16",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5242366",
"TIME": "02:13 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329206751050",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "17",
"NET_AMOUNT": "1751",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "11",
"STAN_NO": "222",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 06:13:02",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5242326",
"TIME": "02:09 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329206751049",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "10",
"STAN_NO": "267",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 06:09:38",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5242250",
"TIME": "02:03 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329206751048",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "9",
"STAN_NO": "261",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 06:03:54",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5242242",
"TIME": "02:02 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329206751047",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "8",
"STAN_NO": "215",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 06:02:35",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5242230",
"TIME": "01:58 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329205751046",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "7",
"STAN_NO": "214",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 05:58:48",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5242214",
"TIME": "01:56 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329205751045",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "6",
"STAN_NO": "212",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 05:56:55",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5242202",
"TIME": "01:55 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329205751044",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "5",
"STAN_NO": "258",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 05:55:04",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5242190",
"TIME": "01:53 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329205751043",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "4",
"STAN_NO": "256",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 05:53:41",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5242170",
"TIME": "01:52 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329205751041",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "3",
"STAN_NO": "254",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 05:52:30",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5242030",
"TIME": "01:35 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329205751035",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michael Jordan",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "1020",
"NET_AMOUNT": "1520",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "2",
"STAN_NO": "193",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "king size bed 10x12",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 05:35:43",
"BILLING_STREET_NO": "2 Jericho Plz",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "50001",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-19-2023",
"REF_TXN_ID": "5241618",
"TIME": "12:42 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329204751017",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "3100",
"CUSTOM_FEE_AMOUNT": "108",
"NET_AMOUNT": "4442",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "612",
"TRAN_NO": "1",
"STAN_NO": "221",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "0",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 04:42:35",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240778",
"TIME": "09:35 PM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329201751012",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michael Jordan",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "1020",
"NET_AMOUNT": "1520",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "29",
"STAN_NO": "154",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "king size bed 10x12",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-19 01:35:13",
"BILLING_STREET_NO": "2 Jericho Plz",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "50001",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240666",
"TIME": "04:17 PM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329120750333",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michael Jordan",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "1020",
"NET_AMOUNT": "1520",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "28",
"STAN_NO": "147",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "king size bed 10x12",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 20:17:11",
"BILLING_STREET_NO": "2 Jericho Plz",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "50001",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240386",
"TIME": "09:44 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329113751618",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "3300",
"CUSTOM_FEE_AMOUNT": "2645",
"NET_AMOUNT": "7674",
"TIP_AMOUNT": "495",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "27",
"STAN_NO": "168",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 13:44:31",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240382",
"TIME": "09:41 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329113751612",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "1200",
"CUSTOM_FEE_AMOUNT": "42",
"NET_AMOUNT": "2476",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "26",
"STAN_NO": "122",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 13:41:44",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240374",
"TIME": "09:38 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329113751605",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "12200",
"CUSTOM_FEE_AMOUNT": "1380",
"NET_AMOUNT": "16644",
"TIP_AMOUNT": "1830",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "25",
"STAN_NO": "120",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 13:38:05",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240366",
"TIME": "09:32 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329113751595",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "1200",
"CUSTOM_FEE_AMOUNT": "42",
"NET_AMOUNT": "2476",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "24",
"STAN_NO": "167",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 13:32:18",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240362",
"TIME": "09:28 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329113751591",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "1100",
"CUSTOM_FEE_AMOUNT": "1380",
"NET_AMOUNT": "3879",
"TIP_AMOUNT": "165",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "23",
"STAN_NO": "118",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 13:28:06",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240222",
"TIME": "09:03 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329113751566",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "1100",
"CUSTOM_FEE_AMOUNT": "39",
"NET_AMOUNT": "2373",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "22",
"STAN_NO": "150",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 13:03:29",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240194",
"TIME": "09:00 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329113751562",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "1100",
"CUSTOM_FEE_AMOUNT": "39",
"NET_AMOUNT": "2373",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "21",
"STAN_NO": "103",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 13:00:19",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240166",
"TIME": "08:55 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329112751558",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "1200",
"CUSTOM_FEE_AMOUNT": "42",
"NET_AMOUNT": "2476",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "20",
"STAN_NO": "144",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 12:55:22",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240158",
"TIME": "08:54 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329112751557",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "1200",
"CUSTOM_FEE_AMOUNT": "42",
"NET_AMOUNT": "2476",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "19",
"STAN_NO": "99",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 12:54:09",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240146",
"TIME": "08:47 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329112751554",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "1200",
"CUSTOM_FEE_AMOUNT": "42",
"NET_AMOUNT": "2476",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "18",
"STAN_NO": "98",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 12:47:13",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240142",
"TIME": "08:40 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329112751549",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "1200",
"CUSTOM_FEE_AMOUNT": "5865",
"NET_AMOUNT": "8479",
"TIP_AMOUNT": "180",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "17",
"STAN_NO": "97",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 12:40:20",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240130",
"TIME": "08:38 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329112751548",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "1200",
"CUSTOM_FEE_AMOUNT": "575",
"NET_AMOUNT": "3189",
"TIP_AMOUNT": "180",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "16",
"STAN_NO": "143",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 12:38:31",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5240122",
"TIME": "08:37 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329112751547",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "1200",
"CUSTOM_FEE_AMOUNT": "575",
"NET_AMOUNT": "3189",
"TIP_AMOUNT": "180",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "15",
"STAN_NO": "142",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 12:37:25",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5239902",
"TIME": "08:07 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329112751532",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michael Jordan",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "1020",
"NET_AMOUNT": "1520",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "14",
"STAN_NO": "133",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "king size bed 10x12",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 12:07:33",
"BILLING_STREET_NO": "2 Jericho Plz",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "50001",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5239738",
"TIME": "07:48 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329111751528",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michale Sumague",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "1200",
"CUSTOM_FEE_AMOUNT": "42",
"NET_AMOUNT": "2476",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "13",
"STAN_NO": "128",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 11:48:38",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5239598",
"TIME": "07:40 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329111751526",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michale Sumague",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "100",
"CUSTOM_FEE_AMOUNT": "600",
"NET_AMOUNT": "1954",
"TIP_AMOUNT": "20",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "12",
"STAN_NO": "75",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 11:40:50",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5239586",
"TIME": "07:40 AM",
"TIMEZONE": "EST",
"AUTH_CODE": null,
"APPROVAL_CODE": null,
"RRN": "",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "94",
"CARDHOLDER_NAME": "1215 Chairman S Michale Su",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "100",
"CUSTOM_FEE_AMOUNT": "4",
"NET_AMOUNT": "1338",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "12",
"STAN_NO": "73",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "Duplicate transmission",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 11:40:12",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5239482",
"TIME": "07:19 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329111751520",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "1215 Chairman S Michale Su",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "200",
"CUSTOM_FEE_AMOUNT": "7",
"NET_AMOUNT": "1441",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "11",
"STAN_NO": "67",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 11:19:29",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5239326",
"TIME": "07:00 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329111751512",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "1215 Chairman S Michale Su",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "5100",
"CUSTOM_FEE_AMOUNT": "600",
"NET_AMOUNT": "7954",
"TIP_AMOUNT": "1020",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "10",
"STAN_NO": "114",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 11:00:41",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5238738",
"TIME": "06:01 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329110751494",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michale Sumague",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "5000",
"CUSTOM_FEE_AMOUNT": "600",
"NET_AMOUNT": "7834",
"TIP_AMOUNT": "1000",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "9",
"STAN_NO": "79",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 10:01:22",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5238690",
"TIME": "06:00 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329110751493",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michale Sumague",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "1230",
"NET_AMOUNT": "3064",
"TIP_AMOUNT": "100",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "8",
"STAN_NO": "24",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 10:00:05",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5238634",
"TIME": "05:48 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329109751492",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "7",
"STAN_NO": "73",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 09:48:08",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5238266",
"TIME": "05:12 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329109751481",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "6",
"STAN_NO": "1000",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": null,
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": null,
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 09:12:04",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5237746",
"TIME": "03:40 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329107751470",
"TXN_TYPE": "REFUND",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michael Jordan",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "012",
"BASE_AMOUNT": "1",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "1",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "5",
"STAN_NO": "977",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 07:40:37",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5237742",
"TIME": "03:40 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329107751464",
"TXN_TYPE": "REFUND",
"CARD_SCHEME": "",
"PAN": "",
"RESPONSE_CODE": "V1",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "012",
"BASE_AMOUNT": "1",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "1",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "5",
"STAN_NO": "11",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "Invalid card token",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 07:40:26",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5237734",
"TIME": "02:39 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329107751464",
"TXN_TYPE": "REFUND",
"CARD_SCHEME": "",
"PAN": "",
"RESPONSE_CODE": "13",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "012",
"BASE_AMOUNT": "1999999900",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "1999999900",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "0",
"TRAN_NO": "0",
"STAN_NO": "10",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "Invalid amount or amount exceeded",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 07:39:38",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5237726",
"TIME": "03:36 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329107751464",
"TXN_TYPE": "REFUND",
"CARD_SCHEME": "",
"PAN": "",
"RESPONSE_CODE": "V1",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "012",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "5",
"STAN_NO": "9",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "Invalid card token",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 07:36:28",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5237714",
"TIME": "03:36 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329107751467",
"TXN_TYPE": "REFUND",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michael Jordan",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "012",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "4",
"STAN_NO": "8",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 07:36:18",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5237710",
"TIME": "03:36 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329107751466",
"TXN_TYPE": "REFUND",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michael Jordan",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "012",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "3",
"STAN_NO": "973",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 07:36:08",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5237650",
"TIME": "03:31 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329107751464",
"TXN_TYPE": "REFUND",
"CARD_SCHEME": "",
"PAN": "",
"RESPONSE_CODE": "12",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "012",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "0",
"NET_AMOUNT": "500",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "3",
"STAN_NO": "3",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "Original transaction not found",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 07:31:03",
"BILLING_STREET_NO": "",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5237630",
"TIME": "03:30 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "111111",
"APPROVAL_CODE": "111111",
"RRN": "329107751464",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4111 XXXX XXXX 1111",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "Michael Jordan",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "1020",
"NET_AMOUNT": "1520",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "2",
"STAN_NO": "968",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "king size bed 10x12",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 07:30:14",
"BILLING_STREET_NO": "2 Jericho Plz",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "50001",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "CREDIT",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5237618",
"TIME": "03:29 AM",
"TIMEZONE": "EST",
"AUTH_CODE": "818888",
"APPROVAL_CODE": "818888",
"RRN": "329107751463",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4012 XXXX XXXX 8888",
"RESPONSE_CODE": "00",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "1020",
"NET_AMOUNT": "1520",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "1",
"STAN_NO": "965",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "king size bed 10x12",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 07:29:38",
"BILLING_STREET_NO": "2 Jericho Plz",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "50001",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5237590",
"TIME": "03:28 AM",
"TIMEZONE": "EST",
"AUTH_CODE": " ",
"APPROVAL_CODE": " ",
"RRN": " ",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4018 XXXX XXXX 8888",
"RESPONSE_CODE": "EB",
"CARDHOLDER_NAME": "",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "1020",
"NET_AMOUNT": "1520",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "1",
"STAN_NO": "999",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "king size bed 10x12",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "Verification error",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 07:28:54",
"BILLING_STREET_NO": "2 Jericho Plz",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "50001",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
},
{
"CARD_TYPE": "",
"EPI": "2203082193",
"LABEL": null,
"DATE": "10-18-2023",
"REF_TXN_ID": "5237586",
"TIME": "03:28 AM",
"TIMEZONE": "EST",
"AUTH_CODE": " ",
"APPROVAL_CODE": " ",
"RRN": " ",
"TXN_TYPE": "SALE",
"CARD_SCHEME": "Visa",
"PAN": "4018 XXXX XXXX 8888",
"RESPONSE_CODE": "EB",
"CARDHOLDER_NAME": "Michael Jordan",
"DBA_NAME": "Test Merchant",
"INVOICE_NO": null,
"POS_ENTRY_MODE": "812",
"BASE_AMOUNT": "500",
"CUSTOM_FEE_AMOUNT": "1020",
"NET_AMOUNT": "1520",
"TIP_AMOUNT": "0",
"TAX_AMOUNT": "0",
"DEVICE_MODEL": "139",
"BATCH_NO": "611",
"TRAN_NO": "1",
"STAN_NO": "998",
"POS_CONDITION_CODE": "59",
"ORDER_DESCRIPTION": "king size bed 10x12",
"PHONE": "",
"EMAIL": "",
"VT_USERNAME": "",
"DISPLAY_MESSAGE": "Verification error",
"IS_SETTLED": "1",
"REFUND_AMOUNT": null,
"REFUND_COUNT": null,
"IS_AUTH_COMPLETED": "0",
"IS_REVERSAL": "0",
"IS_VOID": "0",
"TXN_ORIG_DATE": "2023-10-18 07:28:08",
"BILLING_STREET_NO": "2 Jericho Plz",
"BILLING_STREET_NAME": "",
"BILLING_UNIT": "",
"BILLING_STATE": "",
"BILLING_ZIPCODE": "50001",
"SHIPPING_STREET_NO": "",
"SHIPPING_STREET_NAME": "",
"SHIPPING_UNIT": "",
"SHIPPING_STATE": "",
"SHIPPING_ZIPCODE": ""
}
],
"status": "SUCCESS"
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory txnfetch Transaction type should be set as txnfetch.
date_filter Numeric 25 Optional 0 or 1 Set the date filter ('' => Recent, 0 => Recent, 1 => Today, 2 => MTD, 3 => Yesterday, 4 => Last Month,
5 => Current Week, 6 => Last Wee
start_date_range Date 10 Optional 2023-10-20 Set the start date of fetch the transaction (Ex: YYYY-MM-DD)
end_date_range Date 10 Optional 2023-10-20 Set the start date of fetch the transaction (Ex: YYYY-MM-DD)
transaction_type Numeric 20 Optional 0,1,2 ASet the transaction type ('' => ALL, 0 => ALL, 1 => SALE, 2 => CASH SALE, 3 => DEBIT SALE, 4 => REFUND,
5 => VOID, 6 => AUTH, 7 => COMPLETION, 8 => REVERSAL, 9 => GIFT CARD, 10 => E-INVOICE, 11 =>
PAYNOW, 12 => WITHDRAWAL, 13 => RECURRING, 14 => PURCHASE, 15 => RETURN, 16 => ADVANCE)
card_type Numeric 25 Optional 0,1,2 Set the card brand ('' => ALL, ALL => ALL, 0 => ALL, 1 => VISA, 2 => MASTER CARD, 3 => DISCOVER,
4 => AMEX, 5 => JCB, 6 =>DINERS, 7 => MAESTRO, 8 => EBT, 9 => UNKNOWN CARD)
transaction_status String 25 Optional All, Approved, Declined To fetch the transaction list
devices String 25 Optional ValorPos, VP100 Set the Device type for ('' =>ALL, ALL => ALL, 0 => ALL, 1 => RCKT, 2 => ValorPos 100,
3 => ValorPos 110, 4 => ValorPos 500, 5 => Virtual Terminal, 6 => VP100, 7 => VP500)
filter String 25 Optional EPI, RRN Set the filter options (('' => 0, EPI => EPI, RRN => RRN, MID => MID, AMOUNT => Amount,
APPROVAL_CODE => Approval Code, VT_INVOICE => VT-Invoice, ADDNL_VAL1 => Add Val1, ADDNL_VAL2 => Add Val2, SUBSCRIPTION_ID => Subscription Id))
filter_text String 50 Optional Ex: invoice, 200 To retrieve a transaction by filtering using a text value, you can use the appropriate API.
limit Numeric 10 Mandatory Ex: 200 Limit for pagnation. Max 50 and default 0.
offset Numeric 1 Mandatory Ex: 0 Offset for pagination. Default 0
processor String 25 Optional Valutec, Tsys Sierra Set the processor ('' => ALL, 0 => ALL, 1 => Valutec, 2 => Factor 4, 3 => POSTILION,
4 => EPX , 5 => EPI, 6 => PRIORITY, 7 => AGILPAY, 8 => TSYS Sierra,
9 => FDR Omaha, 10 => FDR CARDNET, 11 => FDR BUYPASS, 12 => FDR NASHVILLEField
Void API
Voiding a payment typically means canceling a payment transaction before it is processed or settled. This action is often taken to prevent the payment from being completed, and the funds are usually returned to the payer's account, making it as if the payment never occurred.
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?void=', [
'body' => '{"appid":"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw","appkey":"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8","epi":"2203082193","txn_type":"void","surchargeindicator":1,"sale_refund":1,"rrn":"329305750774","auth_code":"111111","tran_no":1,"stan":476,"ref_txn_id":"5246234","amount":5,"phone":5248798722,"email":"[email protected]"}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?void="
payload = {
"appid": "WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw",
"appkey": "53PWdki5U0PGSVhRnVoFsfVSbuDutsA8",
"epi": "2203082193",
"txn_type": "void",
"surchargeindicator": 1,
"sale_refund": 1,
"rrn": "329305750774",
"auth_code": "111111",
"tran_no": 1,
"stan": 476,
"ref_txn_id": "5246234",
"amount": 5,
"phone": 5248798722,
"email": "[email protected]"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#fkfd1tylnog14l9');
sdk.voidApi1({
appid: 'WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw',
appkey: '53PWdki5U0PGSVhRnVoFsfVSbuDutsA8',
epi: '2203082193',
txn_type: 'void',
surchargeindicator: 1,
sale_refund: 1,
rrn: '329305750774',
auth_code: '111111',
tran_no: 1,
stan: 476,
ref_txn_id: '5246234',
amount: 5,
phone: 5248798722,
email: '[email protected]'
}, {void: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?void=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"void\",\"surchargeindicator\":1,\"sale_refund\":1,\"rrn\":\"329305750774\",\"auth_code\":\"111111\",\"tran_no\":1,\"stan\":476,\"ref_txn_id\":\"5246234\",\"amount\":5,\"phone\":5248798722,\"email\":\"[email protected]\"}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?void=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"void\",\"surchargeindicator\":1,\"sale_refund\":1,\"rrn\":\"329305750774\",\"auth_code\":\"111111\",\"tran_no\":1,\"stan\":476,\"ref_txn_id\":\"5246234\",\"amount\":5,\"phone\":5248798722,\"email\":\"[email protected]\"}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?void="
payload := strings.NewReader("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"void\",\"surchargeindicator\":1,\"sale_refund\":1,\"rrn\":\"329305750774\",\"auth_code\":\"111111\",\"tran_no\":1,\"stan\":476,\"ref_txn_id\":\"5246234\",\"amount\":5,\"phone\":5248798722,\"email\":\"[email protected]\"}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?void=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"void\",\"surchargeindicator\":1,\"sale_refund\":1,\"rrn\":\"329305750774\",\"auth_code\":\"111111\",\"tran_no\":1,\"stan\":476,\"ref_txn_id\":\"5246234\",\"amount\":5,\"phone\":5248798722,\"email\":\"[email protected]\"}"
response = http.request(request)
puts response.read_body
{
"error_no": "S00",
"error_code": "00",
"amount": "5.00",
"msg": "APPROVED",
"desc": "APPROVAL 111111 ",
"additional_info": null,
"epi": "2203082193",
"channel": "ECOMM",
"rrn": "329305750774",
"txnid": "5246238"
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory void Transaction type should be set as void
amount Numeric 10 Mandatory 100.50 Transaction amount (Maximum amount 99,999.99)
surchargeIndicator Numeric 1 Mandatory 0 or 1 The value '0' represents a transaction being ran on the traditional MID with no custom fee added, while
'1' represents a transaction being ran on the cash discounting MID with the custom fee added
sale_refund Numeric 1 Mandatory 1 Should be set as value 1
auth_code String 8 Mandatory TAS12455 Approval code response from sale transaction (Alphanumeric)
rrn String 15 Mandatory 32921075112 RRN number from sale txn response (Numeric)
tran_no Numeric 6 Mandatory 12 Should match the original sale tran_no
stan Numeric 6 Mandatory 854 Should match the original sale stan
ref_txn_id String 20 Mandatory 5246234 A unique response transaction id from the response of a sale transaction
phone Number 10 Optional 8965342569 Customer phone number - 10 digit
email String 50 Optional [email protected] Customer email id - Varchar 255
Settlement API
A Payment Settlement Merchant, often referred to as a Payment Service Provider (PSP) or Payment Gateway, is a financial institution or service that acts as an intermediary between merchants (sellers) and the financial networks and banks.
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?settlement=', [
'body' => '{"appid":"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw","appkey":"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8","epi":"2203082193","txn_type":"settlement"}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?settlement="
payload = {
"appid": "WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw",
"appkey": "53PWdki5U0PGSVhRnVoFsfVSbuDutsA8",
"epi": "2203082193",
"txn_type": "settlement"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#fkfd1tylnog14l9');
sdk.settlementApi1({
appid: 'WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw',
appkey: '53PWdki5U0PGSVhRnVoFsfVSbuDutsA8',
epi: '2203082193',
txn_type: 'settlement'
}, {settlement: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?settlement=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"settlement\"}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?settlement=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"settlement\"}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?settlement="
payload := strings.NewReader("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"settlement\"}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?settlement=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"settlement\"}"
response = http.request(request)
puts response.read_body
{
"error_no": "S00",
"error_code": "00",
"amount": "0.00",
"msg": "APPROVED",
"desc": null,
"additional_info": "Empty Batch",
"epi": "2203082193",
"channel": "ECOMM",
"rrn": null,
"txnid": null
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory settlement Transaction type should be set as refund
Capture/Ticket
A Payment Capture API is a programming interface that allows developers to integrate payment capture functionality into their applications or websites. Payment capture refers to the process of finalizing a payment transaction by collecting funds from a customer's payment source, such as a credit card or bank account
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?capture=', [
'body' => '{"appid":"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw","appkey":"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8","epi":"2203082193","txn_type":"capture","surchargeIndicator":1,"amount":15.2,"rrn":"329306750795","auth_code":"111111","tran_no":2,"stan":"488"}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?capture="
payload = {
"appid": "WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw",
"appkey": "53PWdki5U0PGSVhRnVoFsfVSbuDutsA8",
"epi": "2203082193",
"txn_type": "capture",
"surchargeIndicator": 1,
"amount": 15.2,
"rrn": "329306750795",
"auth_code": "111111",
"tran_no": 2,
"stan": "488"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#fkfd1tylnog14l9');
sdk.captureticketApi({
appid: 'WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw',
appkey: '53PWdki5U0PGSVhRnVoFsfVSbuDutsA8',
epi: '2203082193',
txn_type: 'capture',
surchargeIndicator: 1,
amount: 15.2,
rrn: '329306750795',
auth_code: '111111',
tran_no: 2,
stan: '488'
}, {capture: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?capture=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"capture\",\"surchargeIndicator\":1,\"amount\":15.2,\"rrn\":\"329306750795\",\"auth_code\":\"111111\",\"tran_no\":2,\"stan\":\"488\"}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?capture=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"capture\",\"surchargeIndicator\":1,\"amount\":15.2,\"rrn\":\"329306750795\",\"auth_code\":\"111111\",\"tran_no\":2,\"stan\":\"488\"}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?capture="
payload := strings.NewReader("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"capture\",\"surchargeIndicator\":1,\"amount\":15.2,\"rrn\":\"329306750795\",\"auth_code\":\"111111\",\"tran_no\":2,\"stan\":\"488\"}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?capture=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"53PWdki5U0PGSVhRnVoFsfVSbuDutsA8\",\"epi\":\"2203082193\",\"txn_type\":\"capture\",\"surchargeIndicator\":1,\"amount\":15.2,\"rrn\":\"329306750795\",\"auth_code\":\"111111\",\"tran_no\":2,\"stan\":\"488\"}"
response = http.request(request)
puts response.read_body
{
"error_no": "S00",
"success_url": true,
"error_code": "00",
"amount": "15.20",
"tax": "",
"customfee": "",
"msg": "APPROVED",
"desc": "APPROVAL 111111 ",
"additional_info": null,
"clerk_id": null,
"clerk_name": null,
"clerk_label": null,
"additionalKeyOne": null,
"additionalKeyTwo": null,
"additionalValueOne": null,
"additionalValueTwo": null,
"approval_code": "111111",
"rrn": "329306750795",
"txnid": "5246582",
"tran_no": 2,
"stan": 488,
"is_partial_approve": 0,
"partial_amount": "000000001520",
"pan": "XXXX",
"card_type": null,
"phone_number": null,
"email_id": null,
"zip": null,
"card_holder_name": null,
"expiry_date": "/",
"address": null,
"epi": "2203082193",
"channel": "ECOMM",
"token": null,
"card_brand": null,
"netamt": 15.2
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory capture Transaction type should be set as capture
amount Numeric 10 Mandatory 100.50 Transaction amount (Maximum amount 99,999.99)
tran_no Numeric 6 Mandatory 12 Should match the original sale tran_no
stan Numeric 6 Mandatory 854 Should match the original sale stan
rrn String 15 Mandatory 32921075112 RRN number from sale txn response (Numeric)
auth_code String 8 Mandatory TAS12455 Approval code response from sale transaction (Alphanumeric)
surchargeIndicator Numeric 1 Mandatory 0 or 1 The value '0' represents a transaction being ran on the traditional MID with no custom fee added, while
'1' represents a transaction being ran on the cash discounting MID with the custom fee added
TIP Adjust
We are considering the implementation of a 'TIP adjust' feature in the payment gateway, allowing users to conveniently adjust tips during transactions.
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?tipAdjust=', [
'body' => '{"appid":"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw","appkey":"r5adF6p4VscO0sLF4NLuNTb9lfBGMGbj","epi":"2313185814","amount":5,"txn_type":"tipadjust","stan":"541","rrn":"5478794578","auth_code":"TA1547"}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?tipAdjust="
payload = {
"appid": "WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw",
"appkey": "r5adF6p4VscO0sLF4NLuNTb9lfBGMGbj",
"epi": "2313185814",
"amount": 5,
"txn_type": "tipadjust",
"stan": "541",
"rrn": "5478794578",
"auth_code": "TA1547"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#fkfd1tylnog14l9');
sdk.tipAdjustApi({
appid: 'WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw',
appkey: 'r5adF6p4VscO0sLF4NLuNTb9lfBGMGbj',
epi: '2313185814',
amount: 5,
txn_type: 'tipadjust',
stan: '541',
rrn: '5478794578',
auth_code: 'TA1547'
}, {tipAdjust: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?tipAdjust=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"r5adF6p4VscO0sLF4NLuNTb9lfBGMGbj\",\"epi\":\"2313185814\",\"amount\":5,\"txn_type\":\"tipadjust\",\"stan\":\"541\",\"rrn\":\"5478794578\",\"auth_code\":\"TA1547\"}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?tipAdjust=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"r5adF6p4VscO0sLF4NLuNTb9lfBGMGbj\",\"epi\":\"2313185814\",\"amount\":5,\"txn_type\":\"tipadjust\",\"stan\":\"541\",\"rrn\":\"5478794578\",\"auth_code\":\"TA1547\"}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?tipAdjust="
payload := strings.NewReader("{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"r5adF6p4VscO0sLF4NLuNTb9lfBGMGbj\",\"epi\":\"2313185814\",\"amount\":5,\"txn_type\":\"tipadjust\",\"stan\":\"541\",\"rrn\":\"5478794578\",\"auth_code\":\"TA1547\"}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?tipAdjust=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"WaJeJErcv5xpqZa2UZz6LZod5MSyyfJw\",\"appkey\":\"r5adF6p4VscO0sLF4NLuNTb9lfBGMGbj\",\"epi\":\"2313185814\",\"amount\":5,\"txn_type\":\"tipadjust\",\"stan\":\"541\",\"rrn\":\"5478794578\",\"auth_code\":\"TA1547\"}"
response = http.request(request)
puts response.read_body
{
"error_no": "S00",
"success_url": true,
"error_code": "00",
"amount": "5.00",
"tax": "",
"customfee": "",
"msg": "APPROVED",
"desc": null,
"additional_info": null,
"clerk_id": null,
"clerk_name": null,
"clerk_label": null,
"additionalKeyOne": null,
"additionalKeyTwo": null,
"additionalValueOne": null,
"additionalValueTwo": null,
"approval_code": "111111",
"rrn": "331306751849",
"txnid": null,
"tran_no": 12,
"stan": 73,
"is_partial_approve": 0,
"partial_amount": "000000000500",
"pan": "XXXX",
"card_type": null,
"phone_number": null,
"email_id": null,
"zip": null,
"card_holder_name": null,
"expiry_date": "/",
"address": null,
"epi": "2203082193",
"channel": "VT",
"token": null,
"card_brand": null,
"netamt": 5
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory tipadjust Transaction type should be set as tipadjust
amount Numeric 10 Mandatory 100.50 Transaction amount (Maximum amount 99,999.99)
stan Numeric 6 Mandatory 854 Should match the original sale stan
rrn String 15 Mandatory 32921075112 RRN number from sale txn response (Numeric)
auth_code String 8 Mandatory TAS12455 Approval code response from sale transaction (Alphanumeric)
Open Batch API
An "Open Batch Payment Gateway" likely refers to a payment gateway that supports batch processing of transactions in an open and accessible manner. In the context of payment processing, batch processing involves the submission and processing of multiple transactions as a group.
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?OpenBatch=', [
'body' => '{"appid":"8301f99aa26536037481546bf5543536","store_id":"15607","appkey":"r5adF6p4VscO0sLF4NLuNTb9lfBGMGbj","txn_type":"openbatch","epi":"2313185814","limit":20,"offset":0}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?OpenBatch="
payload = {
"appid": "8301f99aa26536037481546bf5543536",
"store_id": "15607",
"appkey": "r5adF6p4VscO0sLF4NLuNTb9lfBGMGbj",
"txn_type": "openbatch",
"epi": "2313185814",
"limit": 20,
"offset": 0
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#fkfd1tylnog14l9');
sdk.openBatchApi({
appid: '8301f99aa26536037481546bf5543536',
store_id: '15607',
appkey: 'r5adF6p4VscO0sLF4NLuNTb9lfBGMGbj',
txn_type: 'openbatch',
epi: '2313185814',
limit: 20,
offset: 0
}, {OpenBatch: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?OpenBatch=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"8301f99aa26536037481546bf5543536\",\"store_id\":\"15607\",\"appkey\":\"r5adF6p4VscO0sLF4NLuNTb9lfBGMGbj\",\"txn_type\":\"openbatch\",\"epi\":\"2313185814\",\"limit\":20,\"offset\":0}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?OpenBatch=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"8301f99aa26536037481546bf5543536\",\"store_id\":\"15607\",\"appkey\":\"r5adF6p4VscO0sLF4NLuNTb9lfBGMGbj\",\"txn_type\":\"openbatch\",\"epi\":\"2313185814\",\"limit\":20,\"offset\":0}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?OpenBatch="
payload := strings.NewReader("{\"appid\":\"8301f99aa26536037481546bf5543536\",\"store_id\":\"15607\",\"appkey\":\"r5adF6p4VscO0sLF4NLuNTb9lfBGMGbj\",\"txn_type\":\"openbatch\",\"epi\":\"2313185814\",\"limit\":20,\"offset\":0}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?OpenBatch=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"8301f99aa26536037481546bf5543536\",\"store_id\":\"15607\",\"appkey\":\"r5adF6p4VscO0sLF4NLuNTb9lfBGMGbj\",\"txn_type\":\"openbatch\",\"epi\":\"2313185814\",\"limit\":20,\"offset\":0}"
response = http.request(request)
puts response.read_body
{
"status": "SUCCESS",
"statusMsg": "Open batch request success",
"batchSummary": [
{
"dashboard_netamount": 54773,
"dashboard_batchcount": 8
}
],
"batchSummaryDetails": [
{
"tip_adjusted_count": 2,
"txn_id": 5476054,
"invoice_mode": null,
"epi_id": "2313185814",
"message_type": "0200",
"processing_code": "000000",
"txn_type": "SALE",
"txn_type_code": 101,
"amount": 500,
"tip_amount": 1000,
"cashback_amount": 0,
"custom_fee_amount": 1000,
"isnew_txn": 0,
"surcharge_fee_amount": 0,
"merchant_fee_amount": 0,
"tax_amount": 0,
"tip_fee_amount": 0,
"tax_fee_amount": 0,
"city_tax_amount": 0,
"state_tax_amount": 0,
"original_amount": 0,
"tran_no": 9,
"stan_no": "942",
"invoice_no": "12",
"batch_no": "13",
"pos_entry_mode": "812",
"masked_card_no": "4111 XXXX XXXX 1111",
"card_scheme": "Visa",
"request_date": "11-09-2023",
"request_time": "12:25 AM",
"store_time_zone": "EST",
"mid": "887000003193",
"tid": "75021674",
"rrn": "331305500931",
"approval_code": "TAS230",
"card_menu_selection": "1",
"response_code": "00",
"switch_response_code": "00",
"settlement_mode": null,
"settled_at": "2023-11-09T05:25:02.000Z",
"batches_id": null,
"surver_answer": null,
"additional_doc1": null,
"is_voided": 0,
"is_auth_completed": 0,
"created_at": "2023-11-09T05:25:02.000Z",
"txamount": 2500,
"surcharge_label": "Card Difference"
}
],
"epiInfo": {
"tip_adjust": true,
"max_tip": "350"
}
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
store_id String 12 Mandatory 45258 Merchant store id
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory openbatch Transaction type should be set as openbatch
amount Numeric 10 Mandatory 100.50 Transaction amount (Maximum amount 99,999.99)
limit Numeric 0 Mandatory 0 Limit for pagnation. Max 50 and default 0.
offset Numeric 10 Mandatory 10 Offset for pagination. Default 0
Closed Batch API
A "Closed Batch" in the context of a payment gateway typically refers to a finalized set of transactions that have been collected and are ready for settlement. Once a batch is closed, no additional transactions can be added to it. The closure of a batch is often a step in the settlement process where the payment gateway compiles all the authorized transactions and prepares them for financial settlement.
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?closedBatch=', [
'body' => '{"appid":"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN","appkey":"Tv26MihzxwblHyk6gEuVelY2XwQE58jU","txn_type":"closedbatch","epi":"2319916062","storeid":"20139","batchNO":"17","limit":20,"offset":0}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?closedBatch="
payload = {
"appid": "Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN",
"appkey": "Tv26MihzxwblHyk6gEuVelY2XwQE58jU",
"txn_type": "closedbatch",
"epi": "2319916062",
"storeid": "20139",
"batchNO": "17",
"limit": 20,
"offset": 0
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#fkfd1tylnog14l9');
sdk.closedBatchApi1({
appid: 'Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN',
appkey: 'Tv26MihzxwblHyk6gEuVelY2XwQE58jU',
txn_type: 'closedbatch',
epi: '2319916062',
storeid: '20139',
batchNO: '17',
limit: 20,
offset: 0
}, {closedBatch: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?closedBatch=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"Tv26MihzxwblHyk6gEuVelY2XwQE58jU\",\"txn_type\":\"closedbatch\",\"epi\":\"2319916062\",\"storeid\":\"20139\",\"batchNO\":\"17\",\"limit\":20,\"offset\":0}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?closedBatch=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"Tv26MihzxwblHyk6gEuVelY2XwQE58jU\",\"txn_type\":\"closedbatch\",\"epi\":\"2319916062\",\"storeid\":\"20139\",\"batchNO\":\"17\",\"limit\":20,\"offset\":0}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?closedBatch="
payload := strings.NewReader("{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"Tv26MihzxwblHyk6gEuVelY2XwQE58jU\",\"txn_type\":\"closedbatch\",\"epi\":\"2319916062\",\"storeid\":\"20139\",\"batchNO\":\"17\",\"limit\":20,\"offset\":0}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?closedBatch=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"Tv26MihzxwblHyk6gEuVelY2XwQE58jU\",\"txn_type\":\"closedbatch\",\"epi\":\"2319916062\",\"storeid\":\"20139\",\"batchNO\":\"17\",\"limit\":20,\"offset\":0}"
response = http.request(request)
puts response.read_body
{
"status": "SUCCESS",
"statusMsg": "Closed batch transaction details",
"transactionCount": 20,
"closedBatchDetails": [
{
"txn_id": 5446706,
"epi_id": "2319916062",
"message_type": "0200",
"processing_code": "000000",
"txn_type": "SALE",
"txn_type_code": 101,
"amount": 10000,
"tip_amount": 0,
"cashback_amount": 0,
"custom_fee_amount": 300,
"surcharge_fee_amount": 0,
"merchant_fee_amount": 0,
"tax_amount": 0,
"city_tax_amount": 0,
"state_tax_amount": 300,
"original_amount": 0,
"tran_no": 1,
"stan_no": "403",
"invoice_no": "1",
"batch_no": "17",
"pos_entry_mode": "012",
"masked_card_no": "4111 XXXX XXXX 1111",
"card_scheme": "Visa",
"request_date": "2023-11-01T00:00:00.000Z",
"request_time": "08:32:48",
"mid": "887000003193",
"tid": "75021674",
"rrn": "330512501292",
"approval_code": "TAS110",
"response_code": "00",
"switch_response_code": "00",
"settlement_mode": null,
"settled_at": "2023-11-01T12:32:48.000Z",
"batches_id": null,
"card_menu_selection": "1",
"surver_answer": null,
"additional_doc1": null,
"created_at": "2023-11-01T12:32:48.000Z",
"txamount": 10600,
"is_voided": 1,
"is_auth_completed": 0,
"tip_fee_amount": 0,
"tax_fee_amount": 0
},
]
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
store_id String 12 Mandatory 45258 Merchant store id
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory closedbatch Transaction type should be set as closedbatch
limit Numeric 0 Mandatory 0 Limit for pagnation. Max 50 and default 0.
offset Numeric 10 Mandatory 10 Offset for pagination. Default 0
batchID Numeric 11 Optional 457825 Batch Id for Merchant (Numeric - Ex: 33876)
batchNO Numeric 11 Mandatory 22 Batch number of the open batch details (Numeric - Ex: 54876)
Gift Sale API
The Gift Cards API allows for the sale, activation, check balance and add balance inquiry of gift cards. This API enables the secure and seamless handling of gift card transactions, for products or services offered for sale on their website.
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?giftSale=', [
'body' => '{"appid":"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN","appkey":"1gypMtf5A053r7qcaCpav3G8I4Wbv86i","epi":"2319916601","txn_type":"giftsale","amount":5,"card_no":"7319520000019131778","pin":59008061,"email":"[email protected]"}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?giftSale="
payload = {
"appid": "Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN",
"appkey": "1gypMtf5A053r7qcaCpav3G8I4Wbv86i",
"epi": "2319916601",
"txn_type": "giftsale",
"amount": 5,
"card_no": "7319520000019131778",
"pin": 59008061,
"email": "[email protected]"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#j2ygn1qlqatfga7');
sdk.saleApi2({
appid: 'Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN',
appkey: '1gypMtf5A053r7qcaCpav3G8I4Wbv86i',
epi: '2319916601',
txn_type: 'giftsale',
amount: 5,
card_no: '7319520000019131778',
pin: 59008061,
email: '[email protected]'
}, {giftSale: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?giftSale=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftsale\",\"amount\":5,\"card_no\":\"7319520000019131778\",\"pin\":59008061,\"email\":\"[email protected]\"}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?giftSale=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftsale\",\"amount\":5,\"card_no\":\"7319520000019131778\",\"pin\":59008061,\"email\":\"[email protected]\"}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?giftSale="
payload := strings.NewReader("{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftsale\",\"amount\":5,\"card_no\":\"7319520000019131778\",\"pin\":59008061,\"email\":\"[email protected]\"}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?giftSale=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftsale\",\"amount\":5,\"card_no\":\"7319520000019131778\",\"pin\":59008061,\"email\":\"[email protected]\"}"
response = http.request(request)
puts response.read_body
{
"error_no": "S00",
"success_url": true,
"error_code": "00",
"amount": "5.00",
"tax": "",
"customfee": "",
"msg": "APPROVED",
"desc": null,
"additional_info": null,
"clerk_id": null,
"clerk_name": null,
"clerk_label": null,
"additionalKeyOne": null,
"additionalKeyTwo": null,
"additionalValueOne": null,
"additionalValueTwo": null,
"approval_code": "000000",
"rrn": "004039000001",
"txnid": "5948588",
"tran_no": 2,
"stan": 1571,
"is_partial_approve": 0,
"partial_amount": "000000000500",
"pan": "XXXX1778",
"card_type": null,
"phone_number": null,
"email_id": "[email protected]",
"zip": null,
"card_holder_name": null,
"expiry_date": "/",
"address": null,
"epi": "2319916601",
"channel": "VT",
"token": null,
"card_brand": null,
"netamt": 5
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory giftsale Transaction type should be set as giftsale
amount Numeric 10 Mandatory 100.50 Transaction amount (Maximum amount 99,999.99)
card_no Numeric 16 Mandatory 7319520000019131778 Customer gift card number 15 to 19 digits
pin Numeric 10 Optional 59008061 Customer gift card pin number
email String 50 Optional [email protected] Customer email id - Varchar 255
cardholdername String 25 Optional John Smith Name of the cardholder - Alphabet
phone Number 10 Optional 8965342569 Customer phone number - 10 digit
Gift Card Check Balance
Valor provides an API that allows developers to check the balance of gift cards. You can use the Gift Card API endpoint to retrieve the current balance of a gift card.
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?giftBalance=', [
'body' => '{"appid":"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN","appkey":"1gypMtf5A053r7qcaCpav3G8I4Wbv86i","epi":"2319916601","txn_type":"giftbalance","card_no":"7319520000019131778","pin":"59008061"}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?giftBalance="
payload = {
"appid": "Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN",
"appkey": "1gypMtf5A053r7qcaCpav3G8I4Wbv86i",
"epi": "2319916601",
"txn_type": "giftbalance",
"card_no": "7319520000019131778",
"pin": "59008061"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#j2ygn1qlqatfga7');
sdk.addBalance({
appid: 'Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN',
appkey: '1gypMtf5A053r7qcaCpav3G8I4Wbv86i',
epi: '2319916601',
txn_type: 'giftbalance',
card_no: '7319520000019131778',
pin: '59008061'
}, {giftBalance: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?giftBalance=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftbalance\",\"card_no\":\"7319520000019131778\",\"pin\":\"59008061\"}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?giftBalance=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftbalance\",\"card_no\":\"7319520000019131778\",\"pin\":\"59008061\"}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?giftBalance="
payload := strings.NewReader("{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftbalance\",\"card_no\":\"7319520000019131778\",\"pin\":\"59008061\"}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?giftBalance=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftbalance\",\"card_no\":\"7319520000019131778\",\"pin\":\"59008061\"}"
response = http.request(request)
puts response.read_body
{
"error_no": "S00",
"success_url": true,
"error_code": "00",
"amount": "45.00",
"tax": "",
"customfee": "",
"msg": "APPROVED",
"desc": null,
"additional_info": null,
"clerk_id": null,
"clerk_name": null,
"clerk_label": null,
"additionalKeyOne": null,
"additionalKeyTwo": null,
"additionalValueOne": null,
"additionalValueTwo": null,
"approval_code": "000000",
"rrn": "004039013001",
"txnid": null,
"tran_no": 3,
"stan": 1619,
"is_partial_approve": 0,
"partial_amount": "000000000000",
"pan": "XXXX1778",
"card_type": null,
"phone_number": null,
"email_id": null,
"zip": null,
"card_holder_name": null,
"expiry_date": "/",
"address": null,
"epi": "2319916601",
"channel": "VT",
"token": null,
"card_brand": null,
"netamt": 45
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory giftbalance Transaction type should be set as giftbalance
card_no Numeric 16 Mandatory 7319520000019131778 Customer gift card number 15 to 19 digits
pin Numeric 10 Optional 59008061 Customer gift card pin number
Gift Card Add Balance
With Valor, can add balance to a gift card using the Update Gift Card endpoint. You would send a request to update the gift card with the desired amount to add to the balance.
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://securelink-staging.valorpaytech.com:4430/?addBalance=', [
'body' => '{"appid":"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN","appkey":"1gypMtf5A053r7qcaCpav3G8I4Wbv86i","epi":"2319916601","txn_type":"giftaddvalue","amount":10,"card_no":"7319520000019131778","pin":59008061}',
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?addBalance="
payload = {
"appid": "Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN",
"appkey": "1gypMtf5A053r7qcaCpav3G8I4Wbv86i",
"epi": "2319916601",
"txn_type": "giftaddvalue",
"amount": 10,
"card_no": "7319520000019131778",
"pin": 59008061
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#j2ygn1qlqatfga7');
sdk.addBalance1({
appid: 'Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN',
appkey: '1gypMtf5A053r7qcaCpav3G8I4Wbv86i',
epi: '2319916601',
txn_type: 'giftaddvalue',
amount: 10,
card_no: '7319520000019131778',
pin: 59008061
}, {addBalance: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?addBalance=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftaddvalue\",\"amount\":10,\"card_no\":\"7319520000019131778\",\"pin\":59008061}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?addBalance=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftaddvalue\",\"amount\":10,\"card_no\":\"7319520000019131778\",\"pin\":59008061}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?addBalance="
payload := strings.NewReader("{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftaddvalue\",\"amount\":10,\"card_no\":\"7319520000019131778\",\"pin\":59008061}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?addBalance=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftaddvalue\",\"amount\":10,\"card_no\":\"7319520000019131778\",\"pin\":59008061}"
response = http.request(request)
puts response.read_body
{
"error_no": "S00",
"success_url": true,
"error_code": "00",
"amount": "10.00",
"tax": "",
"customfee": "",
"msg": "APPROVED",
"desc": null,
"additional_info": null,
"clerk_id": null,
"clerk_name": null,
"clerk_label": null,
"additionalKeyOne": null,
"additionalKeyTwo": null,
"additionalValueOne": null,
"additionalValueTwo": null,
"approval_code": "000000",
"rrn": "004039016004",
"txnid": "5948618",
"tran_no": 3,
"stan": 1572,
"is_partial_approve": 0,
"partial_amount": "000000001000",
"pan": "XXXX1778",
"card_type": null,
"phone_number": null,
"email_id": null,
"zip": null,
"card_holder_name": null,
"expiry_date": "/",
"address": null,
"epi": "2319916601",
"channel": "VT",
"token": null,
"card_brand": null,
"netamt": 10
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
amount Numeric 10 Mandatory 100.50 Transaction amount (Maximum amount 99,999.99)
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory giftaddvalue Transaction type should be set as giftaddvalue
card_no Numeric 16 Mandatory 7319520000019131778 Customer gift card number 15 to 19 digits
pin Numeric 10 Optional 59008061 Customer gift card pin number
Gift Card Activate API
Gift Cards API allows you to activate a gift card using the Create Gift Card endpoint. You would provide the necessary information, such as the gift card ID and the initial balance, to create and activate the gift card.
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?activate=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftactive\",\"card_no\":\"7319520000019131778\",\"pin\":\"59008061\"}"
response = http.request(request)
puts response.read_body
import requests
url = "https://securelink-staging.valorpaytech.com:4430/?activate="
payload = {
"appid": "Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN",
"appkey": "1gypMtf5A053r7qcaCpav3G8I4Wbv86i",
"epi": "2319916601",
"txn_type": "giftactive",
"card_no": "7319520000019131778",
"pin": "59008061"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const sdk = require('api')('@valorapi/v1.0#j2ygn1qlqatfga7');
sdk.activate({
appid: 'Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN',
appkey: '1gypMtf5A053r7qcaCpav3G8I4Wbv86i',
epi: '2319916601',
txn_type: 'giftactive',
card_no: '7319520000019131778',
pin: '59008061'
}, {activate: ''})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://securelink-staging.valorpaytech.com:4430/?activate=");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(hnd, CURLOPT_POSTFIELDS, "{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftactive\",\"card_no\":\"7319520000019131778\",\"pin\":\"59008061\"}");
CURLcode ret = curl_easy_perform(hnd);
using RestSharp;
var options = new RestClientOptions("https://securelink-staging.valorpaytech.com:4430/?activate=");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddJsonBody("{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftactive\",\"card_no\":\"7319520000019131778\",\"pin\":\"59008061\"}", false);
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://securelink-staging.valorpaytech.com:4430/?activate="
payload := strings.NewReader("{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftactive\",\"card_no\":\"7319520000019131778\",\"pin\":\"59008061\"}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
require 'uri'
require 'net/http'
url = URI("https://securelink-staging.valorpaytech.com:4430/?activate=")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request.body = "{\"appid\":\"Vw7RdW6IPmRH4Z2feCE8Pp1iqeahSonN\",\"appkey\":\"1gypMtf5A053r7qcaCpav3G8I4Wbv86i\",\"epi\":\"2319916601\",\"txn_type\":\"giftactive\",\"card_no\":\"7319520000019131778\",\"pin\":\"59008061\"}"
response = http.request(request)
puts response.read_body
{
"error_no": "E98",
"error_code": "VR",
"success_url": false,
"txnid": null,
"switch_error_code": "V00VR",
"mesg": "ALREADY ACTIVE",
"desc": "Error code :VR",
"msg": "ALREADY ACTIVE"
}
Field Type Length Mandatory/Optional Example Value Description
appid String 32 Mandatory 464DA39FCFB44D54F6C1D22CEF9098E5 Application id, an unique id given for the application
appkey String 32 Mandatory 15B8BCFDB337428792608354A1444050 Authorization key (API key) given for application contact [email protected]
epi Numeric 10 Mandatory 2536419865 EPI is an end point identifier, Identify the device on valor infrastructure, it's a 10 digit number starts with 2
txn_type String 50 Mandatory giftactive Transaction type should be set as giftactive
card_no Numeric 16 Mandatory 7319520000019131778 Customer gift card number 15 to 19 digits
pin Numeric 10 Optional 59008061 Customer gift card pin number